Implementing Trapezoidal Rule & Simpson's Rule for Numerical Integration in MATLAB
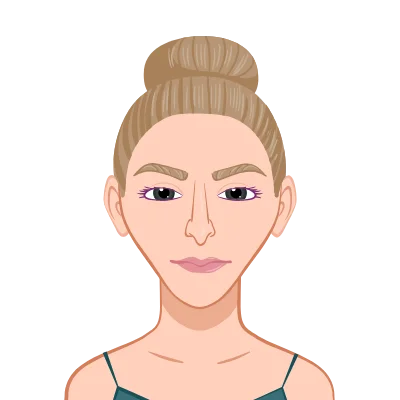
Numerical integration is a fundamental concept in mathematics and computer science, with wide-ranging applications in various fields. This blog aims to provide university students with a theoretical understanding of how to complete your Numerical Integration assignment by implementing the Trapezoidal Rule and Simpson's Rule for numerical integration using MATLAB. We'll explore the fundamental principles behind these methods and their step-by-step implementation in MATLAB.
What is Numerical Integration?
Numerical integration, also known as numerical quadrature, is a technique used to approximate the definite integral of a function. It is particularly valuable when dealing with functions that cannot be easily integrated analytically. Numerical integration methods break the integral into smaller, more manageable pieces and approximate the integral by summing the areas of these pieces.
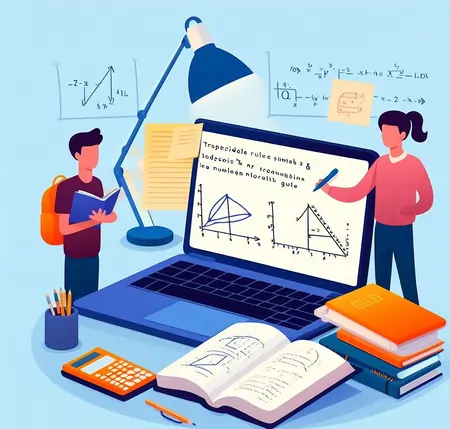
Trapezoidal Rule
The Trapezoidal Rule is a straightforward numerical integration method. It approximates the area under a curve by dividing it into multiple trapezoids. The basic idea is to use trapezoids to estimate the integral of a function.
Steps to Implement the Trapezoidal Rule in MATLAB
The steps for implementing the Trapezoidal rule in MATLAB are:
Step 1: Discretize the Interval
The first step in implementing the Trapezoidal Rule is to discretize the interval [a, b]. Discretization means breaking down the interval into smaller subintervals. This is done to make the approximation more accurate. To achieve this:
- Imagine the interval [a, b] as a line segment.
- Divide it into smaller segments by choosing equally spaced points along the x-axis.
- These points will form the boundaries of the subintervals.
Think of it like slicing a cake into equal pieces. The more slices (subintervals) you have, the closer your approximation will be to the actual integral value.
Step 2: Calculate Function Values
Once you've divided the interval into subintervals, the next step is to calculate the values of the function you want to integrate at each of these points. This involves:
- Taking each of the x-values from the previous step.
- Plugging these x-values into the function f(x) you want to integrate.
- This gives you a set of corresponding y-values, which represent the function's values at those points.
You're essentially finding the heights of the function at each of the chosen x-values. These y-values are crucial for estimating the areas under the curve.
Step 3: Apply the Trapezoidal Rule
Now comes the core of the Trapezoidal Rule. For each subinterval [x_i, x_{i+1}], you approximate the area under the curve by forming a trapezoid. Here's how you do it:
- Connect the two function values, f(x_i) and f(x_{i+1}), at the endpoints of the subinterval with a straight line. This line represents the top side of the trapezoid.
- Draw vertical lines from the endpoints down to the x-axis. These lines represent the two sides of the trapezoid.
- What you now have is a trapezoid formed between the curve and the x-axis within that subinterval.
- The area of this trapezoid is calculated using the formula: (h/2) * (f(x_i) + f(x_{i+1})), where h is the width of the subinterval (the horizontal distance between x_i and x_{i+1}).
You repeat this process for each subinterval in your discretization. Each trapezoid approximates the area under the curve within its subinterval.
Step 4: Sum the Areas
The final step is to sum up the areas of all the trapezoids you obtained in step 3. This summation gives you an approximation of the integral of the function over the entire interval [a, b].
Essentially, you are adding up the areas of all these small trapezoids to estimate the total area under the curve. The more subintervals you use (i.e., the finer the discretization), the more accurate your approximation will be.
In summary, the Trapezoidal Rule in MATLAB involves breaking the interval into smaller pieces, calculating function values at specific points, forming trapezoids to approximate the areas, and finally, summing these areas to estimate the integral. It's a simple but effective method for numerical integration and can be implemented efficiently in MATLAB for various applications in engineering, physics, and other scientific fields.
Simpson's Rule
Simpson's Rule is a more accurate method for numerical integration compared to the Trapezoidal Rule. It approximates the integral by fitting parabolic segments to the curve. This approach provides a better estimate of the integral because it considers the curvature of the function.
Steps to Implement Simpson's Rule in MATLAB
Let's break down the steps to implement Simpson's Rule in MATLAB:
Step 1: Discretize the Interval
Similar to the Trapezoidal Rule, the first step in implementing Simpson's Rule is to break down the interval [a, b] into smaller, more manageable subintervals. To do this:
- Visualize the interval [a, b] as a line segment on the x-axis.
- Divide it into smaller segments by selecting equally spaced points along the x-axis, just like you did with the Trapezoidal Rule.
- These chosen points will serve as the endpoints of your subintervals.
The idea here is to partition the interval into smaller sections to make approximating the integral more accurate.
Step 2: Calculate Function Values
Once you have divided the interval into subintervals, your next task is to calculate the values of the function you want to integrate at each of these chosen points. This involves:
- Taking each of the x-values from the previous step.
- Plugging these x-values into the function f(x) that you are integrating.
- This will yield a set of corresponding y-values, which represent the function's values at those points.
Think of this step as finding the heights of the function at each of the selected x-values. These y-values will be crucial for estimating the areas under the curve.
Step 3: Apply Simpson's Rule
Now, let's get into the heart of Simpson's Rule. For each subinterval [x_i, x_{i+2}], you'll approximate the integral by fitting a quadratic polynomial to the curve within that subinterval. Here's how:
- You connect three function values: f(x_i), f(x_{i+1}), and f(x_{i+2}) with a parabolic curve.
- This curve represents a quadratic polynomial that fits the shape of the function within the subinterval.
The formula for the integral approximation using Simpson's Rule within this subinterval is: (h/3) * (f(x_i) + 4 * f(x_{i+1}) + f(x_{i+2})), where h is the width of the subinterval. This formula takes into account the values of the function at the three points and assigns different weights to them, with the central point (f(x_{i+1})) receiving the highest weight.
Step 4: Sum the Approximations
Finally, to obtain the overall estimate of the integral, you sum up the approximations calculated in Step 3 for all the subintervals.
Think of it like assembling puzzle pieces. Each subinterval contributes its piece of the integral, which is approximated using a quadratic polynomial. When you put all these pieces together (sum them up), you get an estimate of the integral over the entire interval [a, b].
In summary, implementing Simpson's Rule in MATLAB involves dividing the interval into smaller segments, calculating function values at specific points, fitting quadratic polynomials to approximate the areas under the curve, and then summing these approximations to obtain the final estimate of the integral. Simpson's Rule is a more accurate method compared to the Trapezoidal Rule for functions with curvature, and it can be effectively implemented in MATLAB for various scientific and engineering applications.
MATLAB Implementation
In MATLAB, you can implement both the Trapezoidal Rule and Simpson's Rule using a simple loop that follows the steps mentioned above. It's important to choose an appropriate number of subintervals to balance accuracy and computational efficiency. MATLAB provides a flexible environment for this, as it allows you to easily define functions, perform evaluations, and perform iterations.
MATLAB Implementation for Trapezoidal Rule
- Define the Function: Start by defining the function f(x) that you want to integrate. In MATLAB, this is typically done using an anonymous function or by creating a function file.
- Discretize the Interval: Create an array of equally spaced points to divide the interval [a, b] into subintervals. You can use the linspace function in MATLAB to generate these points.
- Calculate Function Values: Use a loop to evaluate the function at each of these points. This loop should iterate through the array of x-values and store the corresponding function values in an array.
- Apply the Trapezoidal Rule: Inside the loop, for each subinterval [x_i, x_{i+1}], calculate the area of the trapezoid using the formula (h/2) * (f(x_i) + f(x_{i+1})), where h is the width of the subinterval.
- Sum the Areas: In the same loop, sum up the areas of the trapezoids as you calculate them. This cumulative sum represents the approximate integral.
- Accuracy Control: You can choose the number of subintervals (i.e., the size of your discretization) to balance accuracy and computational efficiency. A larger number of subintervals will yield a more accurate result but may require more computational resources.
MATLAB Implementation for Simpson's Rule
- Define the Function: Similarly, begin by defining the function f(x) that you want to integrate in MATLAB.
- Discretize the Interval: Create an array of equally spaced points to divide the interval [a, b] into subintervals, just like in the Trapezoidal Rule.
- Calculate Function Values: Use a loop to evaluate the function at each of these points. The loop should iterate through the array of x-values and store the corresponding function values.
- Apply Simpson's Rule: Inside the loop, for each subinterval [x_i, x_{i+2}], calculate the area using the Simpson's Rule formula: (h/3) * (f(x_i) + 4 * f(x_{i+1}) + f(x_{i+2})), where h is the width of the subinterval.
- Sum the Approximations: Similar to the Trapezoidal Rule, sum up the approximations of the integrals within each subinterval as you calculate them.
- Accuracy Control: As with the Trapezoidal Rule, you can control the accuracy of the approximation by choosing an appropriate number of subintervals.
Once you've implemented these steps in MATLAB, you'll have a numerical approximation of the integral of the given function over the specified interval. MATLAB's ability to handle arrays and perform iterative operations makes it a powerful tool for these kinds of numerical computations.
Choosing the Right Rule
When implementing numerical integration in MATLAB, it's crucial to select the right rule for the problem at hand. The Trapezoidal Rule is simple and generally sufficient for functions that don't have significant curvature. However, if your function is smooth and has more complex behavior, Simpson's Rule is a better choice, as it provides a more accurate estimation of the integral.
Conclusion
Numerical integration is a powerful tool for approximating definite integrals of functions, especially when analytical solutions are not readily available. MATLAB offers a user-friendly platform for implementing numerical integration methods like the Trapezoidal Rule and Simpson's Rule. Understanding the theoretical underpinnings of these methods is essential for successful implementation and for making informed decisions about which method to use based on the specific characteristics of the problem at hand.
By following the steps outlined in this blog, university students can gain a strong theoretical foundation and practical knowledge of how to implement these numerical integration methods in MATLAB, thus aiding them in solving assignments and conducting research in various fields of science and engineering.