Enhance Your MATLAB Skills: Building Scripts with User Input and Loops
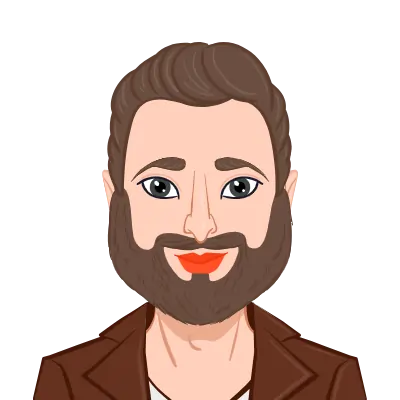
As a student, navigating complex assignments can be challenging. However, with MATLAB, you have a powerful ally that can make these tasks significantly more manageable. In this blog post, we'll explore how to create a MATLAB script that incorporates loops, conditional expressions, and user input to aid you in solving assignments more efficiently. MATLAB offers a user-friendly interface that allows you to focus on problem-solving, making it your perfect assistance with MATLAB assignments. Its extensive documentation and strong online community provide you with the support you need to excel academically.
In this script, we will walk you through solving quadratic equations, a common topic in mathematics and physics assignments. By inputting coefficients and utilizing MATLAB's capabilities, you can swiftly find roots and gain a deeper understanding of the concepts involved. So, let's dive in and see how MATLAB can be your trusted companion in the world of numerical and symbolic computation, providing valuable assistance.
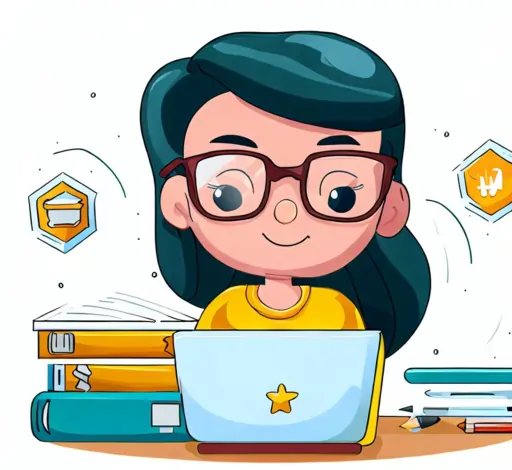
The Power of MATLAB
Before diving into the script, let's briefly discuss why MATLAB is a preferred choice for many students when dealing with assignments related to mathematics, engineering, physics, and data analysis.
- User-Friendly Interface: MATLAB provides an interactive and user-friendly environment that allows students to focus on problem-solving rather than dealing with intricate programming details.
- Comprehensive Documentation: MATLAB offers extensive documentation, a vibrant user community, and numerous online resources, making it easier for students to find solutions to their problems.
- Numerical and Symbolic Computations: It excels in both numerical and symbolic calculations, making it versatile for various academic disciplines.
- Visualization: MATLAB allows students to visualize data and results effectively, which is crucial for understanding complex concepts and presenting findings.
Now, let's move on to creating a MATLAB script that incorporates the elements of loops, conditional expressions, and user input to aid students in their assignments.
The MATLAB Script
In this script, we'll create a simple MATLAB program that calculates the roots of a quadratic equation based on user input. Quadratic equations are a common topic in mathematics and physics assignments, and this script will demonstrate how MATLAB can simplify the process.
Step 1: Gathering User Input
We'll start by prompting the user to input the coefficients (a, b, and c) of the quadratic equation:
% Prompt the user for coefficients
a = input('Enter the coefficient a: ');
b = input('Enter the coefficient b: ');
c = input('Enter the coefficient c: ');
This code uses the input function to collect user input and assigns the values to variables a, b, and c.
Step 2: Calculating the Discriminant
Next, we calculate the discriminant (Δ) of the quadratic equation, which is crucial for determining the nature of the roots:
% Calculate the discriminant
delta = b^2 - 4*a*c;
Step 3: Analyzing the Discriminant
We use conditional expressions to determine the type of roots the quadratic equation has:
if delta > 0
fprintf('The equation has two real and distinct roots.\n');
elseif delta == 0
fprintf('The equation has one real root (a repeated root).\n');
else
fprintf('The equation has two complex roots.\n');
end
This part of the script informs the user about the nature of the roots—real, distinct; real, repeated; or complex—based on the value of the discriminant.
Step 4: Calculating the Roots
Finally, we calculate and display the roots of the quadratic equation:
% Calculate the roots
if delta > 0
root1 = (-b + sqrt(delta)) / (2*a);
root2 = (-b - sqrt(delta)) / (2*a);
fprintf('Root 1 = %.4f\n', root1);
fprintf('Root 2 = %.4f\n', root2);
elseif delta == 0
root = -b / (2*a);
fprintf('Root = %.4f\n', root);
else
realPart = -b / (2*a);
imaginaryPart = sqrt(-delta) / (2*a);
fprintf('Root 1 = %.4f + %.4fi\n', realPart, imaginaryPart);
fprintf('Root 2 = %.4f - %.4fi\n', realPart, imaginaryPart);
end
This part of the script calculates the roots of the quadratic equation based on the nature of the discriminant and displays them to the user.
Putting It All Together
Here's the complete MATLAB script:
% Prompt the user for coefficients
a = input('Enter the coefficient a: ');
b = input('Enter the coefficient b: ');
c = input('Enter the coefficient c: ');
% Calculate the discriminant
delta = b^2 - 4*a*c;
< style="font-size: 0.875rem; color: rgb(0, 0, 0);">% Analyze the discriminant
if delta > 0
fprintf('The equation has two real and distinct roots.\n');
elseif delta == 0
fprintf('The equation has one real root (a repeated root).\n');
else
fprintf('The equation has two complex roots.\n');
end
< style="font-size: 0.875rem; color: rgb(0, 0, 0);">% Calculate and display the roots
if delta > 0
root1 = (-b + sqrt(delta)) / (2*a);
root2 = (-b - sqrt(delta)) / (2*a);
fprintf('Root 1 = %.4f\n', root1);
fprintf('Root 2 = %.4f\n', root2);
elseif delta == 0
root = -b / (2*a);
fprintf('Root = %.4f\n', root);
else
realPart = -b / (2*a);
imaginaryPart = sqrt(-delta) / (2*a);
fprintf('Root 1 = %.4f + %.4fi\n', realPart, imaginaryPart);
fprintf('Root 2 = %.4f - %.4fi\n', realPart, imaginaryPart);
end
How This Script Helps Students
This simple MATLAB script serves as a valuable tool for students in several ways:
- Ease of Use: Students can quickly input the coefficients of a quadratic equation and receive immediate results, simplifying the process of solving such equations.
- Understanding Concepts: By displaying information about the nature of the roots, the script helps students grasp important concepts related to quadratic equations.
- Error Handling: The script handles cases where the discriminant is negative, guiding students through complex roots, which are often tricky to calculate manually.
- Adaptability: This script can be easily adapted for more complex equations or extended to solve a variety of mathematical problems frequently encountered in assignments.
Expanding the Script: Taking It Further
While the quadratic equation solver script we've discussed is a great starting point, MATLAB's capabilities extend far beyond this. Let's explore how we can further enhance the script and introduce additional concepts that can aid students in solving more complex assignments.
1. Error Handling and Input Validation
To make the script more robust, we can add error handling and input validation. For instance, we can ensure that the user inputs are valid numbers and that the equation is indeed quadratic:
% Validate input
if ~isnumeric(a) || ~isnumeric(b) || ~isnumeric(c)
error('Coefficients must be numeric.');
end
if a == 0
error('Coefficient a must not be zero (not a quadratic equation).');
end
These checks prevent the script from proceeding with invalid input, providing helpful error messages to guide students.
2. Graphical Representation
Visualization is a powerful learning tool. We can plot the quadratic equation to help students understand how it behaves:
x = linspace(-10, 10, 400);
% Calculate y values
y = a*x.^2 + b*x + c;
% Plot the quadratic equation
figure;
plot(x, y);
title('Graph of the Quadratic Equation');
xlabel('x');
ylabel('y');
grid on;
% Mark the roots on the plot
hold on;
plot(root1, 0, 'ro', 'MarkerSize', 10);
plot(root2, 0, 'ro', 'MarkerSize', 10);
This addition allows students to visualize how the roots correspond to the points where the graph crosses the x-axis.
3. Generalization for Higher-Order Equations
To extend the script's utility, we can generalize it to handle equations of higher orders, such as cubic or quartic equations. This involves accepting coefficients for all terms and finding roots accordingly. While this requires more complex mathematics, it demonstrates MATLAB's versatility in handling various types of assignments.
4. Optimization and Numerical Techniques
For engineering or scientific assignments, students may need to optimize functions or use numerical techniques like interpolation or integration. MATLAB provides built-in functions and libraries for these tasks, making it a powerful tool for tackling more advanced problems.
5. Simulations and Data Analysis
In assignments related to physics or data analysis, students can benefit from MATLAB's ability to simulate physical systems and analyze data. Incorporating these aspects into scripts can help students gain insights into real-world applications of their coursework.
Resources for Learning MATLAB
Learning MATLAB effectively can significantly benefit students in solving assignments. Here are some resources to get started:
- Official MATLAB Documentation: MATLAB's official documentation is an excellent resource, offering tutorials, examples, and detailed function references.
- Online Courses: Platforms like Coursera, edX, and Udemy offer MATLAB courses covering a wide range of topics and skill levels.
- MATLAB Community: The MATLAB community is active and supportive. MATLAB Central is a hub for discussions, questions, and sharing code.
- Books: There are numerous books dedicated to MATLAB programming and its applications. Some popular options include "MATLAB for Dummies" and "MATLAB Programming for Biomedical Engineers and Scientists."
- University Resources: If you're a student, your university may offer MATLAB workshops or access to MATLAB licenses. Take advantage of these resources.
Conclusion
MATLAB is a versatile tool that can greatly simplify the process of solving assignments in various academic disciplines. By creating scripts that incorporate loops, conditional expressions, and user input, students can enhance their problem-solving skills and gain a deeper understanding of complex concepts. Furthermore, the script can be expanded and customized to suit the specific requirements of different assignments.
As you continue your journey with MATLAB, remember that practice is key to mastering this powerful tool. Experiment with different problems, explore MATLAB's vast library of functions and don't hesitate to seek help from the MATLAB community or your instructors. With dedication and the right resources, MATLAB can be your trusted companion in academic success.