Integrating MATLAB with Python: How to Seamlessly Use Both in Your Assignments
When it comes to programming for engineering, data science, or scientific computing, MATLAB and Python each have unique strengths. MATLAB is a powerful tool for numerical computation and engineering, offering built-in functionalities for matrix operations, simulations, and visualizations. Python, on the other hand, is known for its flexibility, general-purpose applications, and strong ecosystem of libraries like NumPy, Pandas, and Matplotlib. By integrating MATLAB with Python, students can leverage the best of both worlds in their assignments, accessing MATLAB’s high-performance numerical capabilities while utilizing Python’s extensive libraries and flexibility for data manipulation, machine learning, and web applications. For students needing help with MATLAB assignment, combining these two languages can significantly enhance the quality and depth of their work.
A frequent challenge in coursework and assignments is that some complex numerical computations might be easier in MATLAB, while data preprocessing or machine learning tasks are more convenient in Python. Instead of choosing one over the other, students can combine both.
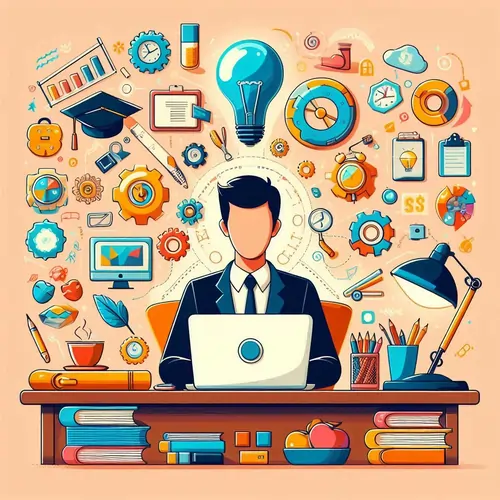
With MATLAB’s Engine API for Python, it is now possible to call MATLAB functions from within a Python script and vice versa. This integration can enhance productivity, streamline workflows, and open new opportunities for tackling assignments that require advanced data processing and visualization.
From a practical standpoint, knowing how to use both MATLAB and Python can also be beneficial for professional development. Many industries use both languages, and familiarity with their integration can give students a competitive advantage in fields like engineering, data science, and research. This guide will explore the steps to integrate MATLAB with Python, outline some use cases, and provide technical insights for getting the most out of this integration in assignments.
Practical Applications of MATLAB and Python Integration for Assignments
Integrating MATLAB with Python can streamline various assignments across different fields. Whether you're tackling tasks in engineering, data science, or research, the ability to combine MATLAB's specialized numerical functions with Python's flexibility opens up new avenues for creativity and problem-solving. Below are a few practical scenarios where this integration can be especially useful for students.
-
Solving Complex Engineering Problems
For engineering students, assignments often require both numerical simulations and advanced mathematical modeling. MATLAB is widely known for its strength in handling complex matrix operations, differential equations, and simulations. Python, however, has a richer ecosystem of tools for handling things like web scraping, machine learning, or automation tasks.
Consider an assignment where you need to simulate the behavior of a mechanical system using MATLAB. Once you have your MATLAB-based model, you can use Python to generate input data from real-world sensors or data sources, preprocess that data, and feed it into your MATLAB model. This could apply to fields like control systems, robotics, or fluid dynamics.
Example:
import numpy as np import matlab.engine # Start the MATLAB engine eng = matlab.engine.start_matlab() # Create input data in Python input_data = np.random.random(100) # Example sensor data # Convert to MATLAB compatible data type matlab_data = matlab.double(input_data.tolist()) # Run the MATLAB model (for example, a control system simulation) result = eng.simulate_system(matlab_data) print(result) eng.quit()
This process allows you to use Python's data-handling power alongside MATLAB's strong engineering libraries, resulting in more efficient and robust solutions to engineering problems.
-
Real-Time Data Analysis and Visualization
Another great use case for integrating MATLAB and Python is when you need to work with real-time data. For example, if you’re handling sensor data from a live experiment or processing streaming data, Python’s libraries (like pandas for data manipulation or matplotlib for preliminary plotting) allow you to handle and analyze the data quickly. Once you have processed the data, you can visualize it in MATLAB for more sophisticated analysis or presentation.
Let’s assume you are tasked with analyzing sensor data from a weather station. The raw data comes in as a time series, which you can clean and analyze with Python’s powerful data manipulation libraries. Afterward, you can pass the data to MATLAB for advanced statistical analysis or 3D visualization.
Example: import pandas as pd import matlab.engine # Start the MATLAB engine eng = matlab.engine.start_matlab() # Load the live weather data in Python data = pd.read_csv("weather_data.csv") temperature_data = data['temperature'].to_numpy() # Convert to MATLAB format and visualize matlab_data = matlab.double(temperature_data.tolist()) eng.plot(matlab_data, nargout=0) eng.title("Temperature Variation") eng.xlabel("Time") eng.ylabel("Temperature (°C)") eng.show() # Stop the engine eng.quit()
This use case helps bridge the gap between real-time data acquisition (Python) and sophisticated analysis and visualization (MATLAB), making your assignments more practical and insightful.
-
Data Science Projects and Machine Learning
Data science students often work with large datasets and employ machine learning algorithms. Python, with its wide array of libraries like Scikit-learn, TensorFlow, and Keras, is a go-to language for machine learning. However, you might still require MATLAB’s specialized tools for certain types of data analysis, like signal processing, image processing, or complex mathematical modeling.
A good example would be using Python to implement and train a machine learning model (like a regression or classification model) and then using MATLAB for deep analysis, model optimization, or visualization of the results.
Example: from sklearn.linear_model import LogisticRegression import matplotlib.pyplot as plt import matlab.engine # Train a model in Python using Scikit-learn model = LogisticRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Visualize in Python first plt.scatter(X_test, y_test, color='blue') plt.plot(X_test, predictions, color='red') plt.title('Logistic Regression Prediction') plt.xlabel('Feature') plt.ylabel('Target') plt.show() # Now pass data to MATLAB for further analysis eng = matlab.engine.start_matlab() matlab_predictions = matlab.double(predictions.tolist()) eng.hist(matlab_predictions, nargout=0) eng.title("Prediction Histogram") eng.show() # Stop the MATLAB engine eng.quit()
Here, you can leverage Python's machine learning libraries for model creation and MATLAB's capabilities for presenting and visualizing the results, making your analysis more thorough and comprehensive.
Steps to Set Up MATLAB and Python Integration
Integrating MATLAB and Python requires some setup to ensure both environments can communicate efficiently. Below, we outline the steps for setting up the integration, assuming you already have both MATLAB and Python installed.
-
Install MATLAB Engine API for Python
To call MATLAB functions from Python, you need to install the MATLAB Engine API for Python. This API allows Python to execute MATLAB commands, retrieve results, and interact with the MATLAB workspace.
- Step 1: Open a terminal (or command prompt) in the directory where MATLAB is installed.
-
Step 2: Run the following command:
cd "matlabroot\extern\engines\python" python setup.py install
- Replace "matlabroot" with the directory where MATLAB is installed. This command installs the necessary libraries for MATLAB and Python integration.
-
Start the MATLAB Engine in Python
After installing the API, you can start MATLAB from within a Python script. Here’s a basic example:
import matlab.engine # Start MATLAB eng = matlab.engine.start_matlab() # Run MATLAB command and get the result result = eng.sqrt(16.0) print(result) # Outputs: 4.0 # Close MATLAB engine eng.quit()
-
Passing Data between MATLAB and Python
A critical part of integrating MATLAB with Python is managing data transfer between the two environments. Python and MATLAB use different data types, so you need to ensure compatibility. The matlab.double() function, for instance, converts Python lists into MATLAB-compatible arrays.
# Example of passing an array from Python to MATLAB data = [1.0, 2.0, 3.0, 4.0] matlab_data = matlab.double(data) result = eng.sum(matlab_data) print(result) # Outputs: 10.0
Use Cases for MATLAB-Python Integration in Assignments
Integrating MATLAB and Python can add significant value to assignments that require both numerical computation and data handling. Here are some specific use cases where this integration can streamline workflows and enhance assignment quality:
-
Case 1: Data Preprocessing in Python, Analysis in MATLAB
Imagine you have an assignment requiring you to analyze a large dataset. Using Python’s Pandas library, you can easily load, clean, and preprocess the data. After preprocessing, you can pass the data to MATLAB for more advanced mathematical analysis or visualization.
import pandas as pd import matlab.engine # Start MATLAB engine eng = matlab.engine.start_matlab() # Load and preprocess data in Python df = pd.read_csv("data.csv") filtered_data = df[df["value"] > threshold].to_numpy() # Pass processed data to MATLAB for analysis matlab_data = matlab.double(filtered_data.tolist()) result = eng.mean(matlab_data) print("Average value:", result) eng.quit()
-
Case 2: Machine Learning in Python, Visualization in MATLAB
Python has extensive libraries like Scikit-Learn for machine learning. However, MATLAB offers excellent visualization tools for engineering data. You can train a model in Python and then visualize the results in MATLAB.
from sklearn.linear_model import LinearRegression
import matlab.engine
# Train a model in Python
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
# Visualize in MATLAB
eng = matlab.engine.start_matlab()
eng.plot(predictions, matlab.double(y_test.tolist()), nargout=0)
eng.title("Prediction vs Actual")
eng.xlabel("Predicted Values")
eng.ylabel("Actual Values")
eng.quit()
This integration not only enriches the functionality of your assignments but also helps you produce insightful visualizations that showcase your analytical skills.
Challenges and Best Practices
While integrating MATLAB and Python can be highly beneficial, it does come with challenges. Performance can be a concern when transferring large datasets between environments. Also, debugging can be more complex since errors can originate from either MATLAB or Python.
To minimize these challenges, consider the following best practices:
- Limit data transfer between MATLAB and Python as much as possible by processing data largely in one environment.
- Use debugging tools available in both languages to isolate errors.
- Document integration steps in your assignments, as this will make it easier for instructors to understand your approach.
Conclusion
Integrating MATLAB and Python can be transformative for students working on assignments that require diverse computational tools. By setting up MATLAB Engine API, you can seamlessly leverage MATLAB's advanced numerical capabilities alongside Python’s powerful libraries, creating a dynamic environment that enhances both computational and visualization power. Whether it’s for data preprocessing, machine learning, or detailed visualizations, this integration expands your toolkit and allows you to approach assignments with a unique, multifaceted perspective.