Implementing the Fast Fourier Transform (FFT) Algorithm in MATLAB
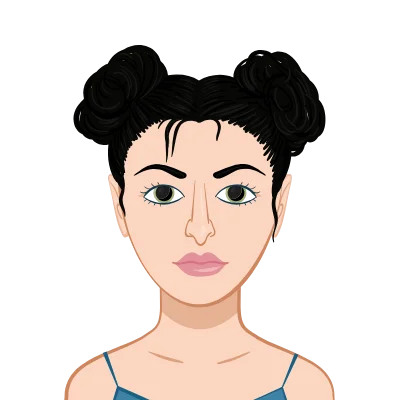
The Fast Fourier Transform (FFT) is a widely used algorithm in various fields such as signal processing, image processing, and data analysis. It is especially valuable for university students who are studying these topics and need to complete your Fast Fourier Transform assignment by implementing the FFT algorithm in MATLAB for efficient computation of discrete Fourier transforms. In this blog, we will provide a theoretical discussion of how to implement the FFT algorithm in MATLAB, without using complex mathematical formulas or code.
What is the Fast Fourier Transform (FFT)?
Before diving into the implementation details, let's briefly understand what the FFT is. The Fast Fourier Transform is an algorithm for computing the Discrete Fourier Transform (DFT) of a sequence or time-domain signal. The DFT represents a signal in the frequency domain, revealing the different frequencies that make up the signal. In MATLAB, you can use the built-in function fft to perform FFT computations.
Key Concepts in FFT Implementation
To implement the Fast Fourier Transform (FFT) algorithm efficiently in MATLAB, it's essential to grasp several key concepts. These concepts form the foundation of the FFT algorithm and are crucial for understanding its theoretical underpinnings. In this section, we will delve deeper into these key concepts without resorting to complex mathematical formulas or code.
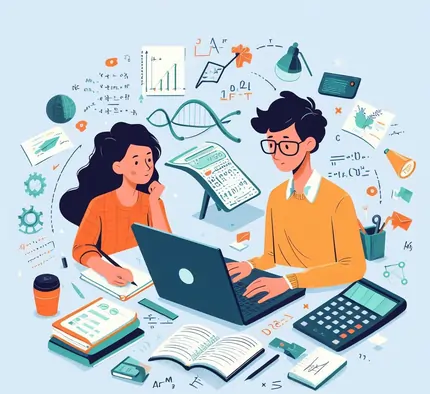
Divide and Conquer
The FFT algorithm employs a "divide and conquer" strategy. This means it breaks down the computation of the Discrete Fourier Transform (DFT) of a sequence into smaller, more manageable subproblems. These subproblems are recursively solved until we reach a base case where the DFT of a single data point is computed.
Think of it as dividing a large problem into smaller, easily solvable chunks. This approach significantly reduces the computational complexity of the DFT calculation.
Butterfly Operation
At the core of the FFT algorithm lies the butterfly operation. This operation is central to combining the results of smaller DFTs to compute the DFT of a larger sequence efficiently. The name "butterfly" comes from the shape of the data flow diagram, which resembles butterfly wings.
A butterfly operation typically involves the following steps:
- Taking two complex numbers as input.
- Performing addition and subtraction between them.
- The results are then combined to produce two new complex numbers.
This process is repeated for each stage of the FFT algorithm and is responsible for the efficient merging of smaller DFTs into a larger DFT.
Radix-2 FFT
The Radix-2 FFT is the most commonly used variant of the FFT algorithm. It is specifically designed to work with sequences whose lengths are powers of 2, such as 2, 4, 8, 16, and so on. The "radix-2" refers to the fact that at each stage of the FFT, the sequence is divided into two smaller sequences.
This choice of radix-2 is essential for achieving efficiency because it simplifies the decomposition process. The Radix-2 FFT is the basis for many FFT implementations, including MATLAB's built-in function, making it highly efficient for practical applications.
Cooley-Tukey Algorithm
The Cooley-Tukey algorithm is a well-known technique for implementing the Radix-2 FFT efficiently. It is named after its developers, J.W. Cooley and John Tukey. This algorithm organizes the computations in a manner that minimizes the number of arithmetic operations required, resulting in faster execution times compared to a straightforward implementation of the DFT.
One of the key features of the Cooley-Tukey algorithm is its ability to factorize the DFT into smaller DFTs. By doing so, it leverages the efficiency of the butterfly operation and optimally exploits the divide-and-conquer strategy.
In summary, understanding these key concepts—divide and conquer, the butterfly operation, Radix-2 FFT, and the Cooley-Tukey algorithm—is crucial for implementing the FFT algorithm efficiently in MATLAB. These principles form the backbone of the FFT algorithm and enable university students to grasp its theoretical foundations, which can be immensely valuable when solving assignments and gaining a deeper understanding of signal processing and data analysis techniques.
Steps to Implement FFT in MATLAB
Implementing the Fast Fourier Transform (FFT) algorithm in MATLAB for efficient computation of discrete Fourier transforms involves a systematic process. In this section, we will outline the steps to guide university students in performing the FFT in MATLAB for assignments and practical applications.
Data Preparation
The first step in implementing the FFT algorithm is to prepare your data. Ensure that your sequence length is a power of 2 (e.g., 2, 4, 8, 16, ...). If your data sequence does not meet this criterion, zero-pad it to the nearest power of 2. Zero-padding involves adding zeros to the end of your data sequence to reach the desired length. This ensures that the FFT algorithm can be efficiently applied to your data.
Radix-2 Decomposition
Once your data is appropriately prepared, proceed to the radix-2 decomposition. Divide your data into smaller sequences, each of length 2. This is the initial step in the "divide and conquer" approach. For example, if your original sequence had a length of 8, you would divide it into four pairs of data points.
Butterfly Operations
The core of the FFT algorithm lies in the butterfly operations. Perform these operations on each pair of sequences at each stage of decomposition. Butterfly operations involve adding and subtracting complex numbers. Keep in mind that these operations are essential for efficiently merging the results of smaller DFTs into a larger DFT. Visualize the data flow as if it's shaped like butterfly wings.
Recursion
Continue the process recursively. As you perform butterfly operations and merge the results, the sequence length will decrease. Continue this process until you reach sequences of length 2. At this point, you can compute the Discrete Fourier Transform (DFT) directly for each pair.
Combine Results
At each stage of recursion, you will obtain intermediate DFT results for smaller sequences. Combine these results by following the butterfly operations until you have computed the DFT of the original, larger sequence. This involves merging the DFTs of smaller sub-sequences into larger sub-sequences until you have the DFT of the entire sequence.
Final Result
After completing all stages of decomposition, combining the results through butterfly operations, and merging sub-sequences, you will arrive at the final result. This final result represents your signal in the frequency domain. It provides insights into the frequency components that make up your original data, which can be useful for various applications such as signal analysis, filtering, and spectral analysis.
The implementation of the Fast Fourier Transform (FFT) algorithm in MATLAB involves a step-by-step process. Beginning with data preparation and proceeding through radix-2 decomposition, butterfly operations, recursion, and result combination, you can efficiently compute the Discrete Fourier Transform (DFT) of your data. This final result represents your signal in the frequency domain, offering valuable insights for university students working on assignments and practical applications in fields such as signal processing, image processing, and data analysis. Understanding these steps is fundamental for mastering the FFT algorithm and its application in MATLAB.
Efficiency Considerations in FFT Implementation
Efficiency is a paramount concern when working with data analysis, particularly when dealing with large datasets. In the context of implementing the Fast Fourier Transform (FFT) algorithm in MATLAB, it's essential to consider efficiency to ensure that computations are performed in a timely manner. Below, we will delve into why efficiency matters and how MATLAB's built-in fft function is optimized for most practical applications. We will also highlight why understanding the underlying principles of the FFT algorithm can be beneficial for debugging and custom applications.
The Importance of Efficiency
Efficiency in FFT implementation is vital for several reasons:
- Speed
- Resource Utilization
- Scalability
- Practicality
Large datasets can be time-consuming to process. An inefficient implementation of the FFT can result in slow computations, which may not be acceptable in time-sensitive applications such as real-time signal processing or data streaming.
Inefficient algorithms can consume excessive memory or processing power, potentially causing system resources to be exhausted. Efficiency ensures that computational resources are used optimally.
As datasets grow in size, the importance of efficiency becomes more pronounced. Efficient algorithms can scale gracefully, allowing you to handle increasingly larger datasets without a significant increase in computation time.
In many real-world applications, you need results quickly. An efficient FFT implementation can make your data analysis workflows more practical and feasible.
MATLAB's Built-in fft Function
MATLAB's built-in fft function is highly optimized for most practical applications. It benefits from years of development and optimization by MATLAB's engineering team. Here are some reasons why you should prefer using fft:
- Speed
- Robustness
- Ease of Use
MATLAB's fft function leverages highly efficient and optimized libraries under the hood. It takes full advantage of hardware acceleration when available, making it one of the fastest FFT implementations for most use cases.
The built-in fft function is thoroughly tested and robust. It can handle a wide range of input data types and sizes without issues.
Using fft is straightforward and doesn't require you to write custom code for common FFT operations. This can save you time and effort in your data analysis tasks.
Understanding Underlying Principles
While MATLAB's fft function is excellent for most scenarios, understanding the underlying principles of the FFT algorithm can still be beneficial:
- Debugging
- Custom Applications
- Learning
In some cases, you might encounter unexpected results or issues with the built-in fft function. Understanding how the FFT works at a fundamental level can help you diagnose and fix these issues.
If you have specific requirements or constraints that MATLAB's fft function cannot address, having a deeper understanding of the FFT algorithm allows you to develop custom implementations tailored to your needs.
For students and researchers, gaining a theoretical understanding of the FFT algorithm is essential for learning and advancing in fields such as signal processing and data analysis. It provides a solid foundation for exploring more advanced topics and algorithms.
Efficiency is a critical consideration when implementing the FFT algorithm, especially for large datasets. MATLAB's built-in fft function is the go-to choice for most practical applications due to its speed, robustness, and ease of use. However, understanding the underlying principles of the FFT algorithm remains valuable for debugging, custom applications, and educational purposes. Balancing the use of optimized tools like fft with a theoretical understanding of the FFT algorithm is key to successful data analysis and signal processing tasks in MATLAB.
Conclusion
The Fast Fourier Transform (FFT) is a powerful algorithm for computing the Discrete Fourier Transform (DFT) of a sequence efficiently. While MATLAB provides a convenient built-in function for FFT computations, it's essential to understand the key concepts of the FFT algorithm, such as divide and conquer, butterfly operations, and Radix-2 decomposition, to implement it effectively for custom applications or assignments.
By following the steps outlined in this blog and grasping the theoretical foundations of the FFT algorithm, university students can gain a deeper understanding of how FFT works and apply it successfully in MATLAB for various signal processing and data analysis tasks.