Revolutionizing Learning through Event-Driven GUI Programming in MATLAB Assignments
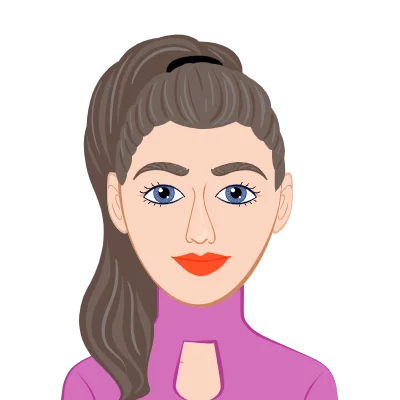
The use of graphical user interfaces (GUIs), which offer a visually intuitive and user-friendly experience, has completely changed how users interact with software applications. GUIs are now essential tools for data analysis, simulations, and educational applications in the MATLAB environment. Event-driven programming is one of the fundamental ideas that underpin the dynamic behavior of MATLAB GUIs. Event-driven programming enables GUIs to react in real-time to user input, enhancing assignments' interactivity and engagement, allowing you to Ace your GUI Assignment. Educators, researchers, and developers can create effective assignments that let students manipulate parameters, visualize data, and actively engage in the learning process by seamlessly integrating event-driven programming into MATLAB GUIs.
Interactive learning experiences have emerged as powerful pedagogical tools as technology continues to change the face of education. It is possible to connect theoretical ideas with real-world applications using MATLAB GUIs. Assignments can move beyond static content and become immersive journeys where students can actively explore concepts, experiment with variables, and see immediate feedback by incorporating event-driven programming. Event-driven MATLAB GUIs enable students to take an active role in their educational journey, encouraging curiosity, critical thinking, and a deeper understanding of the subject matter, whether it be a physics simulation, a mathematical model, or a data analysis task. This blog explores the world of event-driven programming in MATLAB GUIs and demonstrates how it transforms assignments, turning learning into a lively and engaging activity.
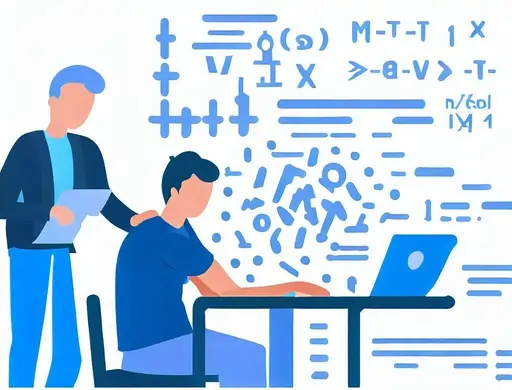
Introduction to Event-Driven Programming in MATLAB GUIs
The use of graphical user interfaces (GUIs) is essential for improving the usability and interactivity of applications. The ability to interact visually with programs makes GUIs in MATLAB a powerful tool for a variety of tasks, including data analysis, simulations, and more. The creation of interactive MATLAB GUIs requires the use of event-driven programming fundamentally. We will examine the idea of event-driven programming in MATLAB GUIs in this blog and learn how it enables interactive assignments. You can create GUIs that respond to user actions and enable dynamic data manipulation by utilizing event-driven programming.
Understanding Event-Driven Programming
Event-driven programming is a paradigm in which a program's flow is decided by outside events rather than by its execution in a linear fashion. These external events primarily include user interactions like mouse clicks, button presses, slider adjustments, and other user-driven actions in the context of MATLAB GUIs. The GUI waits for these events to happen before executing the corresponding event handlers or callbacks, as opposed to iterating through each line of code sequentially.
The event-driven model keeps the GUI in a reactive state so it is always prepared to react to different user actions. For instance, the associated callback function is activated when a user clicks a button, starting the necessary action. By offering real-time responsiveness and interactivity, this design significantly enhances the user experience.
Event-Driven Programming in MATLAB
Utilizing callback functions, event-driven programming is frequently implemented in MATLAB. A user-defined function called a callback is one that is run in response to a specific event. To specify what should happen when a user interacts with a particular GUI component, such as a button, slider, checkbox, or text input, callback functions can be assigned to those components.
In MATLAB, you can use the @(source, event) function_handle syntax to create a callback function. Source denotes the handle of the GUI component that causes the event, and event denotes details about the event itself. The ability to access and change GUI data and properties based on user interactions is made possible by this.
Take into account the following illustration of a MATLAB GUI with a button:
function simpleButtonGUI()
% Create the GUI figure
fig = uifigure('Name', 'Simple Button GUI', 'Position', [100 100 300 200]);
% Create a button
btn = uibutton(fig, 'Position', [100 80 100 40], 'Text', 'Click Me');
% Assign a callback to the button
btn.ButtonPushedFcn = @(source, event) showMessage();
% Function to display a message
function showMessage()
msg = 'Hello, MATLAB GUI!';
uialert(fig, msg, 'Message', 'Icon', 'info');
end
end
When the button is clicked in this example, the callback function showMessage() uses uialert to display a message.
Benefits of Event-Driven Programming in MATLAB GUIs
The use of event-driven programming in MATLAB GUIs has the following benefits:
- Real-time Response: Event-driven GUIs react immediately to user input. By fostering a sense of proximity and interactivity, this capability improves the user experience. Users can change parameters and see real-time updates to the simulation results, for instance, in simulation applications.
- Modularity and Maintainability: Event-driven programming promotes modular code organization and maintainability. The GUI codebase is simpler to maintain and update because each event handler can concentrate on a single task. This division of duties enhances code readability and permits code reuse.
- Efficient Resource Management: Event-driven GUIs use fewer resources because they only run code in response to pertinent events. On the other hand, traditional GUIs may continuously run some processes even if user interaction is not necessary. Event-driven GUIs are more effective as a result, making them appropriate for environments with limited resources.
- User-Friendly Interaction: GUIs with event-driven programming offer an approach that makes it easy for users to interact with sophisticated algorithms and data. Users can alter parameters, see results visualized, and see the results of their actions in real time, which improves learning and gives users the ability to make wise decisions.
Implementing Event-Driven Programming in MATLAB GUIs
Let's start by making a basic GUI with a button that, when clicked, changes the background color of the GUI window to demonstrate event-driven programming in MATLAB.
function simpleGUI()
% Create the GUI figure
fig = uifigure('Name', 'Simple GUI', 'Position', [100 100 300 200]);
% Create a button
btn = uibutton(fig, 'Position', [100 80 100 40], 'Text', 'Click Me');
% Assign a callback to the button
btn.ButtonPushedFcn = @(source, event) changeBackgroundColor(fig);
% Function to change the background color
function changeBackgroundColor(fig)
colors = {'r', 'g', 'b', 'y', 'c', 'm'};
currentColorIndex = mod(numel(fig.Children), numel(colors)) + 1;
fig.Color = colors{currentColorIndex};
end
end
The event handler in this example that adjusts the GUI's background color when the button is pressed is the changeBackgroundColor() function. A predetermined list of colors is cycled through when selecting a color.
Adding Slider Control
Let's expand the functionality of our basic GUI by including a slider that regulates the transparency of a plot that is visible on it.
function sliderControlGUI()
% Create the GUI figure
fig = uifigure('Name', 'Slider Control GUI', 'Position', [100 100 400 300]);
% Create a slider
slider = uislider(fig, 'Position', [150 220 200 3]);
slider.Limits = [0 1];
slider.Value = 0.5;
% Create an axes for plotting
ax = uiaxes(fig, 'Position', [50 50 300 150]);
% Update plot based on slider value
slider.ValueChangedFcn = @(source, event) updatePlot(ax, slider.Value);
% Function to update the plot
function updatePlot(ax, transparency)
x = 0:0.1:10;
y = sin(x);
plot(ax, x, y, 'Color', [0 0.7 0], 'LineWidth', 2, 'Alpha', transparency);
end
end
The event handler for the slider in this example is connected to the updatePlot() function. The transparency of the plot displayed in the GUI is altered in accordance with the value of the slider.
Utilizing Event-Driven Programming in Real-World Applications
In many real-world applications, MATLAB GUIs use event-driven programming. GUIs with event-driven capabilities enable users to interact with and explore data in dynamic ways, from interactive simulations to data visualization tools. Event-driven programming is also used in control systems, where engineers can monitor and change system parameters in real-time thanks to graphical user interfaces (GUIs).
Consider an educational program that allows students to engage with graphic representations of scientific phenomena. Students can adjust the input parameters and watch the simulation's dynamic behavior in real-time by adjusting sliders and buttons. This strategy encourages exploration and curiosity while also improving understanding.
Event-driven GUIs in the field of data analysis enable analysts to interactively explore trends and patterns while visualizing large datasets. Analysts can effectively gain important insights by dynamically changing the filtering criteria or choosing particular data points.
Additionally, event-driven GUIs have a wide range of uses in research, engineering, and other fields where real-time communication and data manipulation are essential for making decisions and solving problems.
Conclusion
In conclusion, event-driven programming in MATLAB GUIs improves application usability and interactivity. GUIs can react instantly to user input by utilizing callback functions and event handlers, enabling dynamic data manipulation and producing more interesting user interfaces. Event-driven GUIs are an indispensable tool for a variety of applications because they are modular, effective, and simple to maintain.
Event-driven programming can enhance the learning experience and make it possible for users to actively engage in the exploration of concepts when creating assignments or educational tools. Users are better able to understand complex concepts when they can interact with simulations, visualize data, and dynamically control parameters. You can design assignments that spark interest, promote learning, and give users a hands-on experience by utilizing the event-driven programming capabilities of MATLAB GUIs.