A Guide to Calculating nth Fibonacci Numbers in MATLAB for Student Assignments
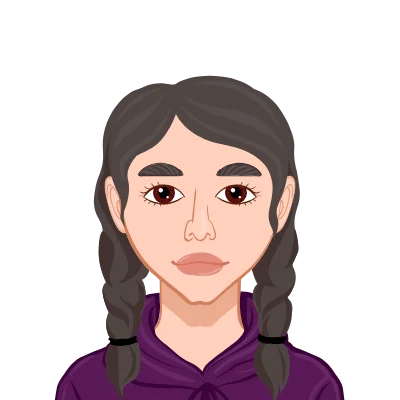
The Fibonacci sequence is a fundamental mathematical concept that has intrigued mathematicians and enthusiasts for centuries. Named after the Italian mathematician Leonardo of Pisa, also known as Fibonacci, this sequence starts with 0 and 1, with each subsequent number being the sum of the two preceding ones. The sequence begins as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on, stretching infinitely.
You might wonder why this seemingly simple sequence holds such importance. Well, the Fibonacci sequence has a wide range of applications across various fields, including mathematics, computer science, biology, and even art. It's found in natural phenomena like the arrangement of leaves on a stem, the spirals of a pinecone, and the proportions in the Parthenon in Athens.
One of the most common assignments for students studying mathematics or computer science is to calculate the nth Fibonacci number. In this blog, we will explore how to write your MATLAB assignment function that efficiently calculates the nth Fibonacci number, helping students tackle this task effectively.
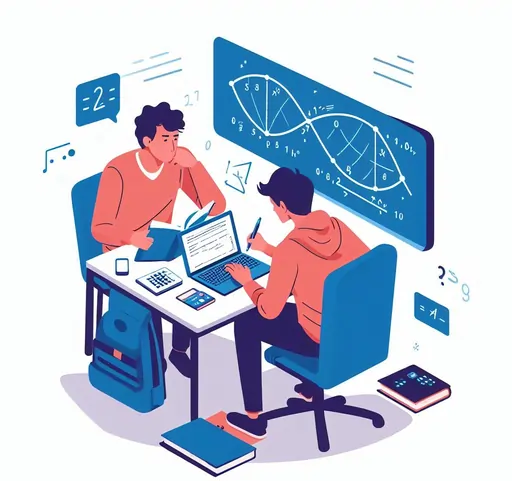
MATLAB: A Powerful Tool for Calculating Fibonacci Numbers
MATLAB (short for MATrix LABoratory) is a versatile programming language and environment used extensively in academia and industry for a wide range of applications. It is particularly well-suited for mathematical and numerical computations, making it an ideal choice for solving problems like calculating Fibonacci numbers.
Before we delve into the code, let's understand the problem-solving approach we'll take:
Problem Statement
Given an integer 'n', we need to find the nth Fibonacci number.
Approach
There are various ways to calculate Fibonacci numbers, but one of the most efficient methods is to use matrix exponentiation. We'll represent the problem in matrix form and use exponentiation to find the nth Fibonacci number. This approach has a time complexity of O(log(n)).
Here's the MATLAB code to achieve this:
function result = fibonacci(n)
% Create a 2x2 matrix representing the Fibonacci transformation
F = [1, 1; 1, 0];
% Check if n is 0; return 0 if true
if n == 0
result = 0;
return;
end
% Calculate F to the power of n-1
Fn = F ^ (n - 1);
% The result is the top right element of Fn
result = Fn(1, 1);
end
This code defines a function fibonacci that takes an integer 'n' as input and returns the nth Fibonacci number. Let's break down how it works:
- We create a 2x2 matrix 'F' that represents the Fibonacci transformation.
- We check if 'n' is 0. If it is, we return 0 since the 0th Fibonacci number is 0.
- We calculate 'Fn' by raising the matrix 'F' to the power of 'n-1'. This is where the matrix exponentiation technique comes into play.
- The result is obtained from the top right element of 'Fn', which is the nth Fibonacci number.
- Using this code, students can efficiently compute Fibonacci numbers, even for large values of 'n'.
Tips for Students and Further Learning
Now that we have a MATLAB function to calculate Fibonacci numbers, here are some tips for students to make the most of this resource and enhance their problem-solving skills:
- Test Your Function
- Analyze Time Complexity
- Explore Alternative Methods
- Apply Fibonacci in Real-World Problems
- Collaborate and Share
Before using the function for any assignments or projects, it's essential to test it with various values of 'n'. Check whether it produces correct results for small values of 'n' that you can manually verify.
Understand the time complexity of the provided function. MATLAB's efficient matrix exponentiation approach has a time complexity of O(log(n)), making it suitable for large values of 'n'. Knowing the time complexity can help you make informed decisions about when and how to use this function.
While the matrix exponentiation method is efficient, it's also valuable to explore other approaches to calculating Fibonacci numbers, such as recursive algorithms or iterative methods. Understanding different methods will broaden your problem-solving skills.
The Fibonacci sequence has applications beyond academic assignments. Explore how it is used in fields like finance, biology, and computer science. You can create your own projects or assignments that involve Fibonacci numbers to gain practical experience.
Don't hesitate to collaborate with peers and share your knowledge. Working together on assignments and discussing different approaches can lead to a deeper understanding of the topic and better problem-solving skills.
Practical Applications of Fibonacci Numbers
Mastering the art of computing Fibonacci numbers through MATLAB transcends mere theoretical pursuits; its practical implications span diverse domains. Delving into tangible situations underscores the tangible benefits of this skill. In the realm of finance, grasping Fibonacci calculations in MATLAB becomes indispensable. Traders rely on Fibonacci retracement levels to make informed decisions about stock market trends, enhancing their strategies and boosting profitability. Likewise, in the field of biology, deciphering Fibonacci numbers aids researchers in modeling population growth and genetic patterns, facilitating breakthroughs in disease control and evolution studies. Let's explore some practical scenarios where the knowledge of Fibonacci numbers and the ability to compute them efficiently can be incredibly useful.
Financial Analysis
Fibonacci numbers have intriguing connections to financial markets and technical analysis. Traders and investors often use Fibonacci retracement levels to identify potential support and resistance levels in stock prices, currencies, and commodities. These retracement levels are calculated based on the Fibonacci sequence. Understanding how to calculate Fibonacci numbers in MATLAB can be valuable for individuals interested in financial markets and technical analysis.
Here's a snippet of code that demonstrates how Fibonacci retracement levels can be calculated in MATLAB:
function retracementLevels = fibonacciRetracement(priceHigh, priceLow)
% Calculate the Fibonacci retracement levels
levels = [0, 0.236, 0.382, 0.500, 0.618, 0.786, 1];
retracementLevels = priceLow + levels * (priceHigh - priceLow);
end
This function takes the highest and lowest prices as input and returns the Fibonacci retracement levels. Traders use these levels to make informed decisions in financial markets.
Bioinformatics
In the field of bioinformatics, Fibonacci numbers play a role in modeling the growth of populations of organisms. The Fibonacci sequence can describe the growth of rabbit populations and the branching of trees. MATLAB is widely used in bioinformatics for data analysis, modeling, and simulation. Students studying bioinformatics can benefit from MATLAB's capabilities to explore Fibonacci-related biological models.
Algorithm Optimization
The efficient calculation of Fibonacci numbers, as shown in the MATLAB function provided earlier, is a testament to the power of algorithm optimization. Understanding and implementing efficient algorithms is a crucial skill for computer scientists and programmers. MATLAB provides a platform for experimenting with different algorithms and analyzing their performance, helping students learn the importance of optimization in solving computational problems.
Art and Design
The Fibonacci sequence and the golden ratio (which is closely related) have long been admired for their aesthetic qualities. They are frequently used in art, architecture, and design to create visually pleasing compositions. MATLAB can be a valuable tool for artists and designers to generate and manipulate Fibonacci-inspired patterns, whether in graphic design, digital art, or even music composition.
Research and Exploration
For students pursuing advanced studies or research, Fibonacci numbers can be a gateway to exploring more complex mathematical concepts. Research projects in number theory, combinatorics, or algorithm design often involve Fibonacci sequences and related mathematical structures. MATLAB's versatility can aid researchers in experimenting with these concepts and developing new insights.
Further Learning and Advanced Applications
Having delved into the practical applications of Fibonacci numbers and grasped their significance, it's time to embark on a more profound journey into the realm of Fibonacci sequences. For those eager to broaden their horizons and enhance their expertise, there are abundant opportunities for advanced learning and applications waiting to be explored. Now that we've uncovered the real-world utility of Fibonacci numbers and comprehended their importance, it's the perfect juncture to take a deeper dive into this mathematical wonder. For individuals with a thirst for knowledge and a desire to elevate their skills, an array of advanced learning avenues and applications beckon.
Advanced Fibonacci Techniques
While the matrix exponentiation method presented earlier is efficient, there are even more advanced techniques for calculating Fibonacci numbers. For example, you can explore closed-form expressions involving the golden ratio, Binet's formula, or even methods involving complex numbers. These advanced techniques can deepen your understanding of the mathematical properties of Fibonacci numbers.
MATLAB Toolbox Integration
MATLAB offers various toolboxes that extend its functionality into specialized domains. For instance, the MATLAB Financial Toolbox provides additional financial analysis capabilities, including tools for working with Fibonacci retracement levels, as mentioned earlier. Exploring these toolboxes can enhance your proficiency in MATLAB and open up career opportunities in specific industries.
Interactive Visualizations
Creating interactive visualizations of Fibonacci-related patterns can be both educational and aesthetically pleasing. MATLAB's graphical capabilities allow you to generate visually engaging plots, animations, and simulations that illustrate Fibonacci concepts. This can be an exciting way to present your findings or teach others about the beauty of mathematics.
Fibonacci Sequences Beyond the Classic
The classic Fibonacci sequence starts with 0 and 1, but what if you want to explore variations or generalizations? MATLAB can be used to compute and analyze other types of Fibonacci-like sequences, such as Lucas numbers, Pell numbers, or Tribonacci numbers. These sequences have their unique properties and applications.
Real-World Projects
To solidify your skills and gain practical experience, consider taking on real-world projects that involve Fibonacci numbers. For example:
- Data Analysis: Analyze datasets where Fibonacci-like patterns may emerge, such as population growth, economic data, or even social media trends.
- Optimization: Use Fibonacci-inspired techniques for optimization problems, such as the Fibonacci search method for finding roots of equations.
- Educational Tools: Develop educational tools or games that use Fibonacci sequences to teach mathematics concepts to students or enthusiasts.
- Artistic Creations: Create digital art, music, or interactive installations that incorporate Fibonacci patterns and principles.
Exploring Advanced MATLAB Techniques for Fibonacci Computation
As you venture further into the realm of MATLAB and Fibonacci numbers, it becomes crucial to broaden your expertise and proficiency. In the upcoming section, we will delve into sophisticated MATLAB methodologies that can elevate your comprehension and aptitude for manipulating Fibonacci sequences. This exploration aims to empower you with advanced tools and insights, facilitating your ability to tackle more complex Fibonacci-related tasks and challenges. By immersing yourself in these advanced techniques, you will be better equipped to harness the full potential of MATLAB in your endeavors with Fibonacci numbers, enabling you to approach intricate sequences with confidence and precision.
Code Optimization
Efficiency is crucial when dealing with large values of 'n' or when working on computationally intensive projects. MATLAB offers several techniques for optimizing code. You can employ profiling tools to identify bottlenecks in your code and make targeted optimizations. Additionally, MATLAB's built-in functions for matrix manipulation can often be leveraged to improve performance further.
Here's an example of code optimization for our Fibonacci function:
function result = fibonacci(n)
% Create a 2x2 matrix representing the Fibonacci transformation
F = [1, 1; 1, 0];
% Check if n is 0; return 0 if true
if n == 0
result = 0;
return;
end
% Calculate F to the power of n-1 using the efficient exponentiation method
Fn = power(F, n - 1);
% The result is the top right element of Fn
result = Fn(1, 1);
end
By using the power function instead of the '^' operator, you can often achieve better performance, especially for large values of 'n'.
Parallel Computing
When dealing with computationally intensive tasks or when you need to calculate Fibonacci numbers for multiple values of 'n' simultaneously, MATLAB's Parallel Computing Toolbox can be a valuable asset. It allows you to harness the power of multi-core processors or distributed computing clusters to speed up your calculations. This can significantly reduce the time required to process large datasets or perform complex simulations.
Interactive User Interfaces
For educational purposes or when developing tools for others, consider creating user-friendly MATLAB graphical user interfaces (GUIs) that allow users to input 'n' and visualize the Fibonacci sequence or related patterns. MATLAB's App Designer provides an intuitive way to design and implement GUIs, making it easier for users to interact with your Fibonacci calculator.
Integration with Other Languages and Tools
MATLAB can be integrated with other programming languages and tools. This can be especially valuable when you need to combine MATLAB's mathematical capabilities with other languages like Python, C++, or Java. Tools like MATLAB Engine APIs allow you to call MATLAB functions from other languages, facilitating seamless integration into larger projects or workflows.
Exploring Big Fibonacci Numbers
As you advance in your studies and projects, you might encounter the need to calculate Fibonacci numbers with very large values of 'n'. MATLAB supports arbitrary-precision arithmetic through the Symbolic Math Toolbox. This toolbox allows you to work with extremely large integers, making it possible to compute Fibonacci numbers that are far beyond the limits of standard numerical data types.
Conclusion
In conclusion, learning how to calculate the nth Fibonacci number in MATLAB is not just about solving assignments; it's a gateway to a world of mathematical exploration and practical applications. The Fibonacci sequence, with its intriguing properties, can be applied in diverse fields, from finance to art, and from biology to computer science.
As a student, the knowledge and skills you acquire while working with Fibonacci numbers in MATLAB can empower you in your academic pursuits and future career endeavors. Whether you're fascinated by the elegance of mathematics, the analytical power of MATLAB, or the real-world impact of Fibonacci applications, this journey offers endless opportunities for growth and innovation.
So, embrace the Fibonacci sequence, dive into MATLAB, and embark on your quest to unravel the mysteries and potential of this timeless mathematical concept. Whether you're a novice or a seasoned MATLAB user, the Fibonacci sequence is a compelling subject that invites exploration, creativity, and endless learning.